Creating Components Dynamically
When using Visual Studio .NET, the Validator component can also be added as a reference. In this case there are a few extra steps you must take, namely:
- You must instantiate your own event handlers.
- You must create and add a licenses.licx file to the project.
Use the following steps to add the Validator component as a reference within Visual Studio .NET:
- Start Visual Studio .NET.
- From the File menu, choose New, then choose Project.
- From the resulting dialog box, choose either Visual C# Projects or Visual Basic Projects then choose Windows Application. Specify a name and a location for the project, then click OK.
- Now add the component as a reference. To do this right-click the References folder in the Solution Explorer and choose Add Reference. If the Solution Explorer is not visible, navigate to the View menu and choose Solution Explorer. The following dialog will appear.
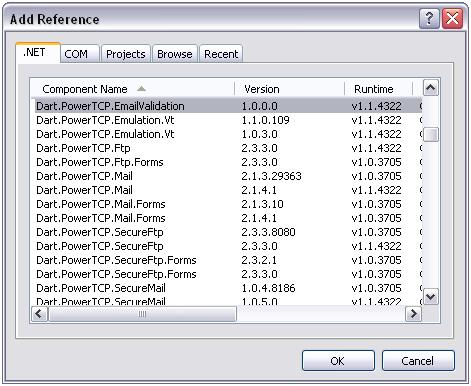
- Select Dart.PowerTCP.Validator.dll and click OK.
- Now you must add a licenses.licx file to the project. (See the topic Creating A "licenses.licx" File" for more information).
- Now make a quick application to test. Drag a button onto the form and insert the following code:
[C#]
using Dart.PowerTCP.Validator;
private void button1_Click(object sender, System.EventArgs e)
{
// Test the syntax of an email address
Validator Validator1 = new Validator();
ValidationState result = Validator1.Validate("myAddress@myDomain.com", ValidationLevel.Syntax);
if (result.Exception == null)
MessageBox.Show("The syntax is valid!");
else
MessageBox.Show(result.Exception.Message);
}
- Navigate to the Debug menu and choose Start. The app will compile and execute. Click the button and you should see a message appear.
- Note: This example shows usage without events. For most applications you will have to create your own event handlers. To do this, see the Using Events topic.
In This Section
- Overview
- This topic provides an overview for using PowerTCP Email Validation for .NET.
- Placing Components on a Form
- This topic demonstrates how to create and use components using Visual Studio .NET.
- Creating Components Dynamically
- This topic demonstrates how to add a component as a reference using Visual Studio .NET.
- Synchronous vs. Asynchronous Use
- This topic discusses the benefits and uses for synchronous and asynchronous methods.
- Using Events
- This topic discusses how to use events with the component, both using Visual Studio .NET and in other environments.
blockEmail Validation Using Menu
Send comments on this topic.
Documentation version 1.0.3.0.
© 2008 Dart Communications. All rights reserved.