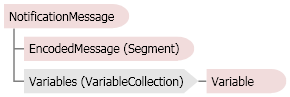
Visual Basic (Declaration) | |
---|---|
Public Class NotificationMessage Inherits StandardMessage |
Visual Basic (Usage) | ![]() |
---|---|
Dim instance As NotificationMessage |
C# | |
---|---|
public class NotificationMessage : StandardMessage |
Managed Extensions for C++ | |
---|---|
public __gc class NotificationMessage : public StandardMessage |
C++/CLI | |
---|---|
public ref class NotificationMessage : public StandardMessage |
The following example demonstrates receiving trap, notification and inform messages.
C# | ![]() |
---|---|
private void button1_Click(object sender, EventArgs e) { //Start listening for traps, notifications and informs manager1.Start(manager1_MessageReceived, null); } private void manager1_MessageReceived(Manager manager, MessageBase message, object state) { //Marshal message to the UI thread using the Message event if (message is TrapMessage) manager.Marshal(new MessageBase[] { message }, "trap"); else if (message is NotificationMessage) manager.Marshal(new MessageBase[] { message }, "notification"); else if (message is InformMessage) { manager.Marshal(new MessageBase[] { message }, "inform"); //Send response to inform message origin manager.Send(new ResponseMessage(message as InformMessage), message.Origin); } } private void manager1_Message(object sender, MessageEventArgs e) { //Update interface according to message type received switch (e.UserState.ToString()) { case "trap": TrapMessage trap = e.Messages[0] as TrapMessage; label1.Text = "Trap received with Enterprise(" + trap.Enterprise + "), Generic Type (" + trap.GenericTrap.ToString() + "), Specific Type(" + trap.SpecificTrap.ToString() + ")"; break; case "notification": NotificationMessage notification = e.Messages[0] as NotificationMessage; label1.Text = "Notification received with OID (" + notification.Oid + ")"; break; case "inform": InformMessage inform = e.Messages[0] as InformMessage; label1.Text = "Inform received with " + inform.Variables.Count.ToString() + " variable(s)."; break; } } |
Visual Basic | ![]() |
---|---|
Private Sub button1_Click(ByVal sender As Object, ByVal e As EventArgs) Handles button1.Click 'Start listening for traps, notifications and informs manager1.Start(AddressOf manager1_MessageReceived, Nothing) End Sub Private Sub manager1_MessageReceived(ByVal manager As Manager, ByVal message As MessageBase, ByVal state As Object) 'Marshal message to the UI thread using the Message event If TypeOf message Is TrapMessage Then manager.Marshal(New MessageBase() {message}, "trap") ElseIf TypeOf message Is NotificationMessage Then manager.Marshal(New MessageBase() {message}, "notification") ElseIf TypeOf message Is InformMessage Then manager.Marshal(New MessageBase() {message}, "inform") 'Send response to inform message origin manager.Send(New ResponseMessage(TryCast(message, InformMessage)), message.Origin) End If End Sub Private Sub manager1_Message(ByVal sender As Object, ByVal e As MessageEventArgs) Handles manager1.Message 'Update interface according to message type received Select Case e.UserState.ToString() Case "trap" Dim trap As TrapMessage = TryCast(e.Messages(0), TrapMessage) label1.Text = "Trap received with Enterprise(" & trap.Enterprise & "), Generic Type (" & _ trap.GenericTrap.ToString() & "), Specific Type(" & trap.SpecificTrap.ToString() & ")" Case "notification" Dim notification As NotificationMessage = TryCast(e.Messages(0), NotificationMessage) label1.Text = "Notification received with OID (" & notification.Oid & ")" Case "inform" Dim inform As InformMessage = TryCast(e.Messages(0), InformMessage) label1.Text = "Inform received with " & inform.Variables.Count.ToString() & " variable(s)." End Select End Sub |
Three classes are included that specialize the NotificationMessage class to standard NOTIFICATION-OBJECTs that are defined in RFC 1907:
1. Dart.Snmp.Notification.coldStart
2. Dart.Snmp.Notification.warmStart
3. Dart.Snmp.Notification.authenticationFailure
The MibManager class can be used to add additional classes that derive from NotificationMessage.
System.Object
Dart.Snmp.MessageBase
Dart.Snmp.StandardMessage
Dart.Snmp.NotificationMessage
Dart.Snmp.Notification.authenticationFailure
Dart.Snmp.Notification.coldStart
Dart.Snmp.Notification.warmStart
Target Platforms: Microsoft .NET Framework 2.0